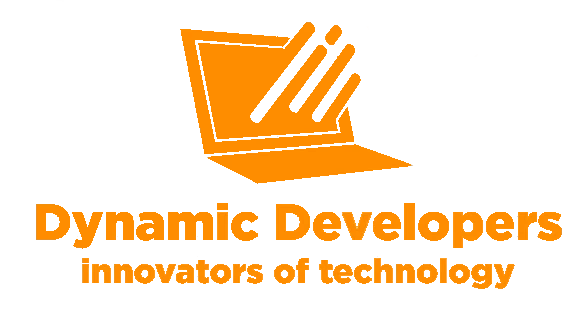
Let's read and know about the different activities and blogs by Dynamic Developers.
Facial detection in computer vision is an important aspect of artificial intelligence and computer science. It involves the process of identifying and locating faces in digital images or videos. This technology is used in a wide range of applications such as security systems, surveillance, and image editing. In this article, we will explore the basics of facial detection in computer vision and provide sample code for implementing this technology.
Facial detection technology involves the use of algorithms that analyze digital images or videos to locate and identify human faces. These algorithms typically use a combination of machine learning techniques, statistical analysis, and pattern recognition to identify and analyze facial features. Facial detection algorithms can be divided into two categories: feature-based detection and template matching.
Feature-based detection algorithms use a set of predefined facial features such as the eyes, nose, and mouth to locate and identify faces in an image. These algorithms analyze the size, position, and shape of these facial features to identify a face. Template matching algorithms, on the other hand, use a pre-existing template or model of a face to match and locate faces in an image. These algorithms analyze the similarities between the template and the image to identify faces.
Sample code for implementing facial detection in computer vision can be written in several programming languages such as Python, Java, and C++. In this article, we will provide a sample code in Python using the OpenCV library.
First, we need to import the OpenCV library in our code. We can do this using the following code:
import cv2
Next, we need to load an image or video that we want to analyze for facial detection. We can do this using the following code:
img = cv2.imread('image.jpg')
Once we have loaded the image, we can use the CascadeClassifier function in OpenCV to create a classifier that can detect faces. We can use the following code to create a face classifier:
face_cascade = cv2.CascadeClassifier('haarcascade_frontalface_default.xml')
The 'haarcascade_frontalface_default.xml' file is a pre-trained model for detecting faces in images. We can download this file from the OpenCV website.
After creating the face classifier, we can use the detectMultiScale function to detect faces in the image. This function returns a list of rectangles that indicate the position and size of each detected face. We can use the following code to detect faces in the image:
faces = face_cascade.detectMultiScale(img, 1.3, 5)
The first parameter of the detectMultiScale function is the image we want to analyze, the second parameter is the scale factor, and the third parameter is the minimum number of neighbors.
Finally, we can draw rectangles around the detected faces in the image using the following code:
for (x,y,w,h) in faces: cv2.rectangle(img,(x,y),(x+w,y+h),(255,0,0),2)
The rectangle function in OpenCV is used to draw a rectangle around the detected face. The first parameter is the image we want to draw on, the second parameter is the starting point of the rectangle, the third parameter is the ending point of the rectangle, the fourth parameter is the color of the rectangle, and the fifth parameter is the thickness of the rectangle.
In conclusion, facial detection in computer vision is a powerful technology that has a wide range of applications. We provided a sample code in Python using the OpenCV library to detect faces in an image. With this basic knowledge of facial detection, developers can explore and experiment with this technology to create more complex applications.